Setup Python Project on MacOS
As a developer, setting up a new project can be a daunting task, especially when it comes to configuring the environment. In this article, we'll walk you through the process of installing and configuring Python for your projects on MacOS.
Install Python 3
The first step is to install Python 3 using Homebrew, a popular package manager for MacOS. Open your terminal and run the following command:
brew install python@3.11
Once installed, verify the version by running:
python3 -V
Install pip
Next, we need to install pip, the package installer for Python. Run the following command:
python3.11 -m pip install --upgrade pip
Set up Virtual Environment (venv)
A virtual environment is a self-contained Python environment that allows you to isolate your project's dependencies from the system-wide Python installation. To set up a virtual environment, run the following commands:
python3.11 -m pip install virtualenv
Sample Project Setup
Now let us create a new directory called demo and set up a virtual environment within it.
mkdir demo
cd demo
python3.11 -m venv env
source env/bin/activate
pip list
pip install --upgrade pip
Install Dependencies
Now that we have our virtual environment set up, let's install the dependencies required for our project. In this case, we need to install urllib3, certifi, and other packages. Run the following commands:
pip install urllib3 certifi
pip freeze > requirements.txt
pip install -r requirements.txt
Sample Code
Let's add some sample code to demonstrate how to read an API endpoint and print the response. Create a new file called demo.py with the following content:
import os
import urllib3
import certifi
import json
api = 'https://api.frankfurter.app'
exc_dt = '2023-10-15'
currency = 'eur'
currency_api = api + '/' + exc_dt + '?from=' + currency
http = urllib3.PoolManager(ca_certs=certifi.where())
response = http.request("GET", currency_api)
jsonResponse = response.json()
print(json.dumps(jsonResponse, indent=2))
Run the Program
Finally, let's run our program by executing the following command:
python demo.py
This will print the API response in JSON format.
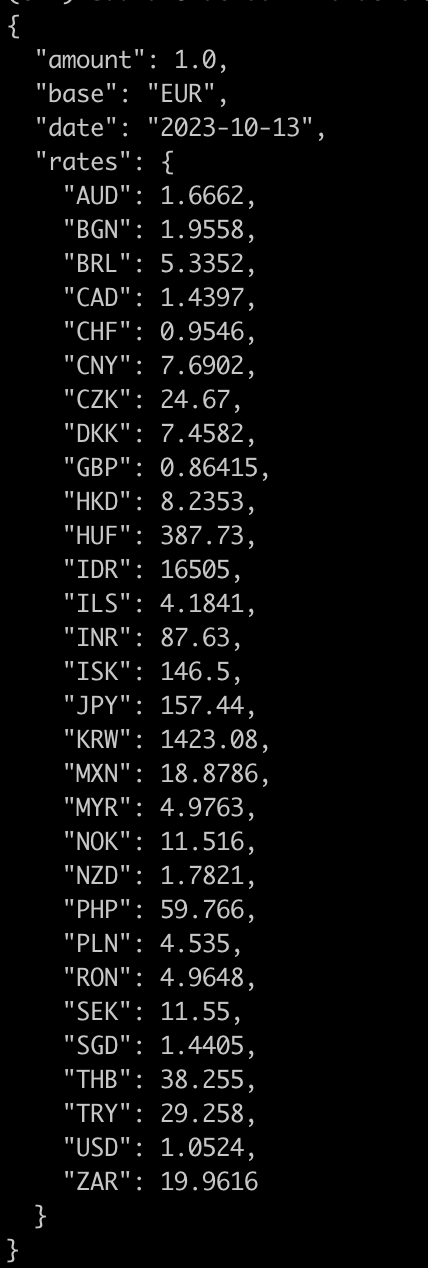
Deactivate Virtual Environment
When you're finished with your project, don't forget to deactivate the virtual environment using the following command:
deactivate
That's it! With these steps, you should have a fully set up Python project on MacOS. Happy coding!